Home » Eclipse Projects » Eclipse Platform » How can I wrap TableViewer cells text?
How can I wrap TableViewer cells text? [message #709133] |
Wed, 03 August 2011 14:33  |
arthurav2005 Messages: 10 Registered: August 2011 |
Junior Member |
|
|
I have a table in which some columns may be smaller than the text. I want the text to wrap on more rows. The default behavior in TableViewer with ColumnLabelProvider is to show ... instead of the remaining text. I have explicitly set SWT.WRAP on each column, but it doesn't do what I want.
The person class used:
package utilclass;
public class Person {
public String first;
public String second;
public String gender;
public Person(String firs, String sec, String gen){
first=firs;
second=sec;
gender=gen;
}
}
The code for the View is the following:
package test2_wrap;
import java.util.ArrayList;
import org.eclipse.jface.viewers.ArrayContentProvider;
import org.eclipse.jface.viewers.ColumnLabelProvider;
import org.eclipse.jface.viewers.TableViewer;
import org.eclipse.jface.viewers.TableViewerColumn;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.GridData;
import org.eclipse.swt.layout.GridLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.TableColumn;
import org.eclipse.ui.part.ViewPart;
import utilclass.Person;
public class View extends ViewPart {
public static final String ID = "test2_wrap.view";
private TableViewer viewer;
private Label label;
private Button button;
private GridData labelData;
private ArrayList<Person> myCol;
public void createPartControl(Composite parent) {
myCol=new ArrayList<Person>();
myCol.add(new Person("a1", "very loooooooong name","m"));
myCol.add(new Person("a2", "y","n"));
viewer = new TableViewer(parent, SWT.MULTI | SWT.H_SCROLL
| SWT.V_SCROLL | SWT.FULL_SELECTION | SWT.BORDER);
createColumns(parent, viewer);
viewer.getTable().setLayout(new GridLayout(2,true));
viewer.getControl().setLayoutData(new GridData(SWT.FILL, SWT.FILL, true, true ));
viewer.getTable().setHeaderVisible(true);
viewer.setContentProvider(new ArrayContentProvider());
viewer.setInput(myCol);
}
private void createColumns(final Composite parent, final TableViewer viewer) {
String[] titles = { "First name", "Last name", "Gender" };
int[] bounds = { 100, 100, 100 };
// First column is for the first name
TableViewerColumn col = createTableViewerColumn(titles[0], bounds[0], 0);
col.setLabelProvider(new ColumnLabelProvider() {
@Override
public String getText(Object element) {
Person p = (Person) element;
return String.valueOf(p.first);
}
});
col = createTableViewerColumn(titles[1], bounds[1], 1);
col.setLabelProvider(new ColumnLabelProvider() {
@Override
public String getText(Object element) {
Person p = (Person) element;
return String.valueOf(p.second);
}});
col = createTableViewerColumn(titles[2], bounds[2], 1);
col.setLabelProvider(new ColumnLabelProvider() {
@Override
public String getText(Object element) {
Person p = (Person) element;
return String.valueOf(p.gender);
}});
}
private TableViewerColumn createTableViewerColumn(String title, int bound, final int colNumber) {
final TableViewerColumn viewerColumn = new TableViewerColumn(viewer,
SWT.CENTER | SWT.WRAP);
final TableColumn column = viewerColumn.getColumn();
column.setText(title);
column.setWidth(bound);
column.setResizable(true);
column.setMoveable(true);
return viewerColumn;
}
public void setFocus() {
viewer.getControl().setFocus();
}
}
Is there a way to change the labelprovider behaviour the "very looooooooong name" on multiple lines instead of diplaying ... ?
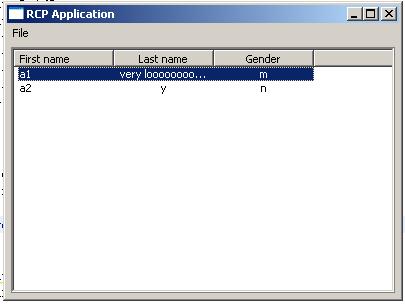
Thank you
[Updated on: Wed, 03 August 2011 14:54] Report message to a moderator
|
|
|
Re: Wrap TableViewer cells text [message #709193 is a reply to message #709133] |
Wed, 03 August 2011 16:02   |
Thomas Schindl Messages: 6651 Registered: July 2009 |
Senior Member |
|
|
You need to use owner draw but even with that you are in trouble because
all rows in a table have to have the same height!
Tom
Am 03.08.11 16:33, schrieb arthurav2005:
> I have a table in which some columns may be smaller than the text. I want the text to wrap on more rows. The default behavior in TableViewer with ColumnLabelProvider is to show ... instead of the remaining text. I have explicitly set SWT.WRAP on each column, but it doesn't do what I want.
>
> The code for the View is the following:
>
>
> package test2_wrap;
>
> import java.util.ArrayList;
>
> import org.eclipse.jface.viewers.ArrayContentProvider;
> import org.eclipse.jface.viewers.ColumnLabelProvider;
> import org.eclipse.jface.viewers.TableViewer;
> import org.eclipse.jface.viewers.TableViewerColumn;
> import org.eclipse.swt.SWT;
> import org.eclipse.swt.layout.GridData;
> import org.eclipse.swt.layout.GridLayout;
> import org.eclipse.swt.widgets.Button;
> import org.eclipse.swt.widgets.Composite;
> import org.eclipse.swt.widgets.Label;
> import org.eclipse.swt.widgets.TableColumn;
> import org.eclipse.ui.part.ViewPart;
>
> import utilclass.Person;
>
> public class View extends ViewPart {
> public static final String ID = "test2_wrap.view";
>
> private TableViewer viewer;
> private Label label;
> private Button button;
> private GridData labelData;
> private ArrayList<Person> myCol;
>
>
> public void createPartControl(Composite parent) {
> myCol=new ArrayList<Person>();
> myCol.add(new Person("a1", "very loooooooong name","m"));
> myCol.add(new Person("a2", "y","n"));
> viewer = new TableViewer(parent, SWT.MULTI | SWT.H_SCROLL
> | SWT.V_SCROLL | SWT.FULL_SELECTION | SWT.BORDER);
> createColumns(parent, viewer);
> viewer.getTable().setLayout(new GridLayout(2,true));
> viewer.getControl().setLayoutData(new GridData(SWT.FILL, SWT.FILL, true, true ));
> viewer.getTable().setHeaderVisible(true);
> viewer.setContentProvider(new ArrayContentProvider());
> viewer.setInput(myCol);
>
> }
>
> private void createColumns(final Composite parent, final TableViewer viewer) {
> String[] titles = { "First name", "Last name", "Gender" };
> int[] bounds = { 100, 100, 100 };
>
> // First column is for the first name
> TableViewerColumn col = createTableViewerColumn(titles[0], bounds[0], 0);
> col.setLabelProvider(new ColumnLabelProvider() {
> @Override
> public String getText(Object element) {
> Person p = (Person) element;
> return String.valueOf(p.first);
> }
>
> });
>
> col = createTableViewerColumn(titles[1], bounds[1], 1);
>
> col.setLabelProvider(new ColumnLabelProvider() {
> @Override
> public String getText(Object element) {
> Person p = (Person) element;
> return String.valueOf(p.second);
> }});
>
> col = createTableViewerColumn(titles[2], bounds[2], 1);
>
> col.setLabelProvider(new ColumnLabelProvider() {
> @Override
> public String getText(Object element) {
> Person p = (Person) element;
> return String.valueOf(p.gender);
> }});
> }
>
> private TableViewerColumn createTableViewerColumn(String title, int bound, final int colNumber) {
> final TableViewerColumn viewerColumn = new TableViewerColumn(viewer,
> SWT.CENTER | SWT.WRAP);
> final TableColumn column = viewerColumn.getColumn();
> column.setText(title);
> column.setWidth(bound);
> column.setResizable(true);
> column.setMoveable(true);
> return viewerColumn;
>
> }
>
> public void setFocus() {
> viewer.getControl().setFocus();
> }
> }
>
>
> Is there a way to change the labelprovider behaviour the "very looooooooong name" on multiple lines instead of diplaying ... ?
>
>
>
> Thank you
|
|
| | | | |
Goto Forum:
Current Time: Fri Apr 26 11:14:38 GMT 2024
Powered by FUDForum. Page generated in 0.03712 seconds
|