Polygon Figure with gradient pattern ,does not paint properly at different zooms. [message #634537] |
Fri, 22 October 2010 07:19 |
Vijay Raj Messages: 608 Registered: July 2009 |
Senior Member |
|
|
I have created a snippet to recreate my problem
package test;
import org.eclipse.draw2d.ColorConstants;
import org.eclipse.draw2d.FigureCanvas;
import org.eclipse.draw2d.Graphics;
import org.eclipse.draw2d.PolygonShape;
import org.eclipse.draw2d.ScalableFreeformLayeredPane;
import org.eclipse.draw2d.geometry.PointList;
import org.eclipse.draw2d.geometry.Rectangle;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.events.SelectionListener;
import org.eclipse.swt.graphics.Pattern;
import org.eclipse.swt.layout.GridData;
import org.eclipse.swt.layout.GridLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
/**
* <dl>
* <dd> <code>Draw2dPatternTest.java</code> TODO: Class Description goes here.</dd>
* </dl>
*
* @author vijay
* @version 1.0.0
*/
public class Draw2dPatternTest
{
public static class CustomPolygonShape extends PolygonShape
{
/**
* @see org.eclipse.draw2d.PolygonShape#shapeContainsPoint(int, int)
*/
@Override
protected boolean shapeContainsPoint(int x, int y)
{
return getPoints().polygonContainsPoint(x, y);
}
/**
* @see org.eclipse.draw2d.PolygonShape#fillShape(org.eclipse.draw2d.Graphics)
*/
@Override
protected void fillShape(Graphics graphics)
{
Pattern pattern = new Pattern(Display.getCurrent(), getBounds().getTopLeft().x,
getBounds().getTopLeft().y, getBounds().getBottomRight().x, getBounds()
.getBottomRight().y, ColorConstants.white, getBackgroundColor());
graphics.setBackgroundPattern(pattern);
graphics.fillPolygon(getPoints());
pattern.dispose();
}
/**
* @see org.eclipse.draw2d.PolygonShape#outlineShape(org.eclipse.draw2d.Graphics)
*/
@Override
protected void outlineShape(Graphics graphics)
{
graphics.drawPolygon(getPoints());
}
}
public static class DiamondFigure extends CustomPolygonShape
{
/** points list for diamond figure. */
private PointList pl;
/**
* @see org.eclipse.draw2d.Figure#setBounds(org.eclipse.draw2d.geometry.Rectangle)
*/
@Override
public void setBounds(Rectangle rect)
{
super.setBounds(rect);
pl = new PointList();
pl.addPoint(bounds.x + bounds.width / 2, bounds.y);
pl.addPoint(bounds.x, bounds.y + bounds.height / 2);
pl.addPoint(bounds.x + bounds.width / 2, bounds.y + bounds.height);
pl.addPoint(bounds.x + bounds.width, bounds.y + bounds.height / 2);
setPoints(pl);
}
}
private static String string;
/**
* @param args
*/
public static void main(String[] args)
{
Display display = new Display();
final Shell shell = new Shell(display);
shell.setLayout(new GridLayout(3, true));
final Button zoomOut = new Button(shell, SWT.PUSH);
zoomOut.setText("Zoom Out");
final Label l = new Label(shell, SWT.None);
Button zoomIn = new Button(shell, SWT.PUSH);
zoomIn.setText("Zoom In");
string = "Zoom Level=";
l.setText(string + "1");
FigureCanvas c = new FigureCanvas(shell);
GridData layoutData = new GridData(GridData.FILL_BOTH);
layoutData.horizontalSpan = 3;
c.setLayoutData(layoutData);
c.setBackground(display.getSystemColor(SWT.COLOR_WHITE));
final ScalableFreeformLayeredPane figure = new ScalableFreeformLayeredPane();
figure.setBackgroundColor(ColorConstants.white);
figure.setForegroundColor(ColorConstants.white);
CustomPolygonShape s = new DiamondFigure();
s.setBounds(new Rectangle(0, 0, 100, 100));
s.setBackgroundColor(ColorConstants.green);
figure.add(s);
c.setContents(figure);
SelectionListener listener = new SelectionListener()
{
double i = 1;
@Override
public void widgetSelected(SelectionEvent e)
{
if (e.getSource() == zoomOut)
{
i += .20d;
figure.setScale(i);
}
else
{
i -= .20d;
figure.setScale(i);
}
l.setText(string + i);
shell.layout();
}
@Override
public void widgetDefaultSelected(SelectionEvent e)
{
// TODO Auto-generated method stub
}
};
zoomOut.addSelectionListener(listener);
zoomIn.addSelectionListener(listener);
shell.open();
while (!shell.isDisposed())
{
if (!display.readAndDispatch()) display.sleep();
}
display.dispose();
}
}
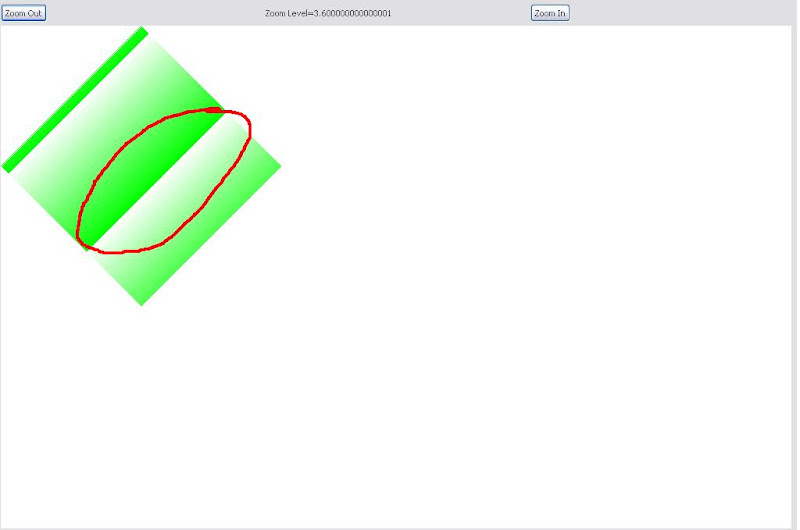
as you can see from the snippet application at higher zoom levels ,the diamond shape patternt painting gets distorted.
My questions are
1.Is the problem OS level.
2.Is it a bug in draw2d.
3.is there any workaround
---------------------
why, mr. Anderson, why, why do you persist?
Because I Choose To.
Regards,
Vijay
|
|
|
Powered by
FUDForum. Page generated in 0.02242 seconds