Express Yourself More by Switching to Java 12 - A Preview of Enablement!
During our times, there lives a very popular and a matter-of-fact person, whose thoughts are balanced, philosophical and well-grounded. Lately though, on looking around, the person finds all the young kids next block having fun with sophisticated gadgets which never existed in earlier times and a desire is born in our protagonist's mind to acquire some of the better, newer gadgets. However, as we all know, our protagonist is a highly-regarded old-school decision maker and doesn't want the name acquired so far to get tainted by being seen in the wrong company - be it of people or of gadgets. After a period of deep thought, the protagonist comes up with this brilliant idea of creating a new temporary identical persona - a persona which will adapt all new and noteworthy latest and greatest gadgets to allow the friends to play with those. Depending on the friendly advices of the near and dear ones about how the persona behaved, our protagonist will adapt these gadgets and the associated behaviors to make those a permanent characteristic.
Phew! That was my miserable attempt on literary creativity; before I make Shakespeare turn in his grave, let me get a little technical.
The protagonist here is of course our good old Java, as you might have guessed already. As you all know, Java is planning to add multiple features in the next few releases. Given that the Java releases a new version every six months now, the duration is not good enough to release features with timely, user-driven feedback. Hence, in the previous version of Java, which is 11, it released an infrastructure, or "persona" if you may, called the "preview feature" support. Preview feature support helps users to try out new features which are "previewed" and provide feedback to Java developers. Depending on the user pulse, the preview feature might be adapted with little or a lot of changes, or even discarded. This facility provides new and exciting features to be incorporated in Java only after proper experimentation and user feedback driven improvements, thus continuing to keep Java sacrosanct while acquiring new features aka "gadgets".
Though "preview feature" was available in the previous release, the "enablement", happened, for all practical purposes with the release of current version of Java, 12, since the first experimental feature came out in Java 12. Let us start with this experimental feature and then figure out how to enable it.
Consider the following source code:
public class Y { enum Day { MON, TUE, WED, THUR, FRI, SAT, SUN }; public String getDay(Day today) { String day = null; switch(today) { case MON: case TUE: day = "Blues"; break; case WED: day = "Hectic"; break; case THUR: case FRI: day = "Getting better"; break; case SAT: case SUN: day = "Life!"; break; }; return day; } }
The code listing above shows how we assign a local string variable based on specific values of enum using a switch statement. For this we use the fall-through of case statements and breaks wherever required and then in the end the value in the local variable is returned. If the whole point is to assign a variable, can we make switch statement itself assign a value to the variable "day"? Hold on, statements do not have values, only expressions do. Hmm.. Then why don't we have "switch" expression as well which has the capability of returning a value? That's exactly the Java 12 gives you. To provide switch expressions, Java 12 overloaded the "break" statement to allow to return a value, for e.g. break "Blues" where the "Blues" is a value and not a label to jump to. That throws up the question for some - How do we figure out whether the right-hand part of break statement is a label or a value - the "value" in the break is valid only in switch expressions - the Eclipse Java Compiler will flag an error if there was an attempt to code otherwise.
public class X { enum Day { MON, TUE, WED, THUR, FRI, SAT, SUN }; public String getDay(Day today) { String day = switch(today) { case MON: case TUE: break "Blues"; case WED: break "Hectic"; case THUR: case FRI: break "Getting Better"; case SAT: case SUN: break "Life!"; }; return day; } }
That was only the first iteration. Let us try to see if there are further improvements: The overdose of "case" statements is an immediate eye-sore for someone who is new to Java and/or who is using some of the newer languages. Java 12 proposes to ease this by allowing multiple labels in a "case" statement thus allowing case MON, TUE instead, for e.g. And now we have the following:
public class X { enum Day { MON, TUE, WED, THUR, FRI, SAT, SUN }; @SuppressWarnings("preview") public String getDay(Day today) { String day = switch(today) { case MON, TUE: break "Blues"; case WED: break "Hectic"; case THUR, FRI: break "Getting Better"; case SAT, SUN: break "Life!"; }; return day; } }
No one is ever satisfied - the same goes for the above as well. Folks felt that the presence of multiple "break" statements is just unnecessary ceremony which can be made implicit. Hence Java 12 overloaded the "->" operator to provide the more concise syntax with the final code transformation as shown below:
public class X { enum Day { MON, TUE, WED, THUR, FRI, SAT, SUN }; public String getDay (Day today) { return switch(today) { case MON, TUE -> "Blues"; case WED -> "Hectic"; case THUR, FRI -> "Getting Better"; default -> "Life!"; }; } }
To try out, just copy and paste the code listing above into Eclipse 4.12 or above.
This will show the "preview" disabled error. Use the quick-fix of "Enable preview features..." to enable. Subsequently, a @SuppressWarnings
("preview") can be added above getDay() to suppress the "preview feature used" warning as well. Essentially, after the preview is enabled, Java Compiler section of (project specific, if you may) configuration will look like the following:
And a final runnable program, complete with SuppressWarnings
directive and a main() is given below:
public class X { enum Day { MON, TUE, WED, THUR, FRI, SAT, SUN }; @SuppressWarnings("preview") public String getDay(Day today) { return switch(today) { case MON, TUE -> "Blues"; case WED -> "Hectic"; case THUR, FRI -> "Getting Better"; default -> "Life!"; }; } public static void main(String[] args) { System.out.println(new X().getDay(Day.MON)); } }
There's just one more detail before we can run a program in preview mode, we need to tell the JVM that the class file is generated with preview enabled and hence the running should also be in the preview enabled mode. This is automatically taken care if you are running within Eclipse; However, if you are planning to use command line JVM to run this class, use "--enable-preview" in the command line.
Before we forget, the fundamental motivation of preview features is to understand user experience and get feedback. So please do rant, complain, appreciate in the JDK mailing lists, or for Eclipse Java Development feature requests or modifications do submit bugs or enhancement requests.
Friends, Switch to Eclipse with Java 12, enable preview and express yourself more using switch expressions, and give feedback to keep Java evergreen! That will be, with due apologies to Neil Armstrong, "A small step for Java users, to enable a giant step for Java"!
About the Author
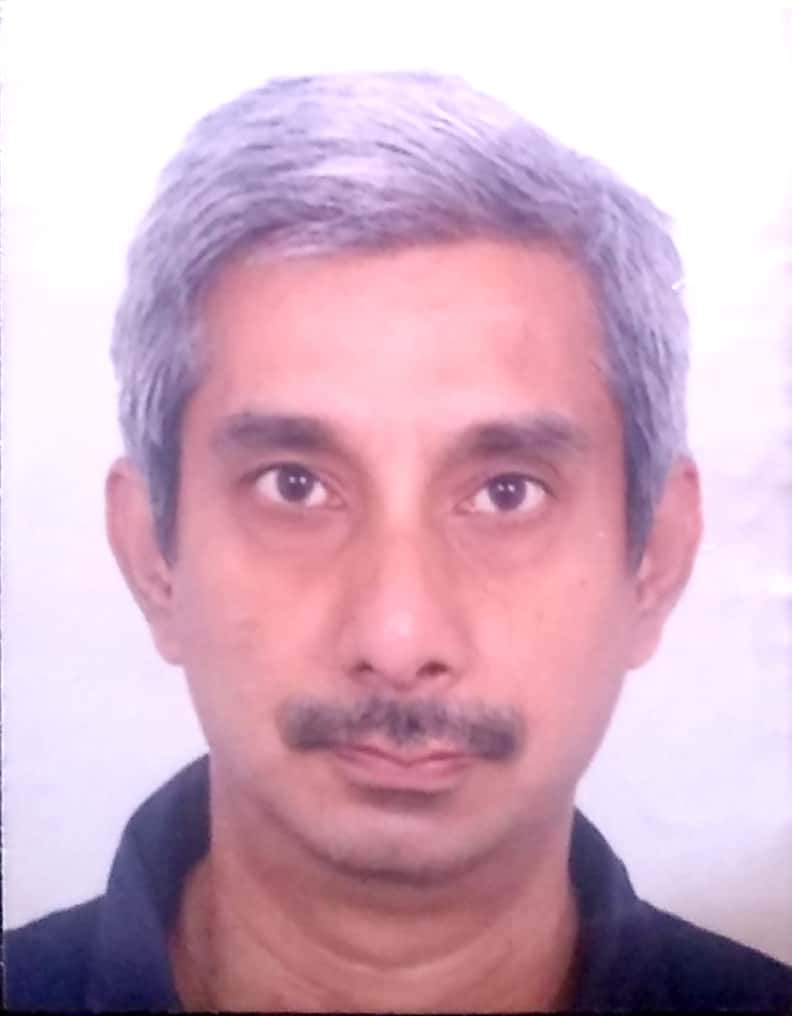