Eclipse RCP and REST: An Introduction
Modular user interfaces, particularly the Eclipse Rich Client Platform (RCP), can help developers leverage the benefits of microservices. The first step is to create the technical infrastructure required to integrate with the backend microservices. In this article, I’ll demonstrate how an Eclipse RCP client can easily consume a service that’s exposed as a REST endpoint.
When demonstrating this approach, it’s helpful to have a stable and interesting REST API to consume. Luckily, SpaceX provides a set of well-documented services that describe various domains — launches, missions, rockets, and many others. In this example, I’ll demonstrate how to consume the SpaceX Launch Service.
I’ve kept the example as simple as possible to clearly show the moving parts. You should be able to clone this repository and get things running very quickly. I’ve also created a repository in GitHub that shows the implementation in more detail.
Setting up the Environment
The approach relies on a set of Eclipse frameworks that work together to make it very simple to consume REST services using Jakarta RESTful Web Services (JAX-RS). Figure 1 shows the stack of these frameworks.
Figure 1: Eclipse Frameworks Stack Used to Consume REST Services
These frameworks allow you to create a Java interface that’s annotated with JAX-RS, then expose a proxy to that interface as an Open Services Gateway initiative (OSGi) service. Apache CXF and Eclipse Jersey implementations are provided, and Jersey is used in the example. Both providers use the Jackson JavaScript Object Notation (JSON) processor to handle data binding between the service and the Java types.
There is a GitHub repository for the JAX-RS providers. If you would like to consume the pre-built features in an Eclipse target definition file or Tycho build, you can use this p2 repository location.
However, if you’re trying to set this up for the first time, I highly recommend using the target definition I’ve included in the GitHub repository.
Describing and Discovering the Service
One way to describe a service is to use an Endpoint Description Extender Format (EDEF) file. An EDEF file describes how to connect to an endpoint and how to map the service to Java types using JAX-RS. The two most important attributes described by an EDEF file are the Uniform Resource Identifier (URI) of the endpoint and the Java type that will represent the service. Figure 2 illustrates these descriptions.
Figure 2: EDEF File Base Endpoint URI and Java Interface Descriptions
To ensure the EDEF file is discovered at runtime, include a Remote-Service entry in your OSGi manifest, as shown below:
Remote-Service: OSGI-INF/LaunchService_EDEF.xml
At runtime, the EDEF file is read and a proxy for the Java interface is registered as an OSGi service. The service can then be called without knowing or caring that a REST service is working behind the scenes.
Implementing the Service
The EDEF file specifies a Java interface annotated using JAX-RS, as shown below.
The launch data returned from the service must also be represented. In the example code shown below, Jackson annotations are used to help with object mapping, but it’s possible to have a completely unannotated Plain Old Java Objects (POJOs) mapping as well.
Running the code at this point would result in a LaunchService instance that is available as an OSGi service. This service could be consumed using regular OSGi mechanisms, such as a Service Tracker or Declarative Services, and used in any OSGi environment. I’ll describe how to access it from an Eclipse RCP application.
Integrating With Eclipse RCP
While an Eclipse RCP client can consume OSGi services using a Service Tracker or Declarative Services, you can also inject services directly into the UI components.
To inject a service created using the JAX-RS provider:
- Inject the service using the @Inject and @Service annotations. The @Service annotation fixes some class-loading issues that occur with the normal injection mechanism.
- Configure the plug-in containing the UI component to be activated when one of its classes is requested. You can do this on the Overview page of the Manifest Editor, as shown in Figure 3.
Figure 3: Manifest Editor Overview Page
The injected service can now be used inside the Eclipse RCP UI component to request SpaceX launch data, as shown below.
In this section of the code, I’m retrieving the launch number and name, then displaying the data in a table. Figure 4 shows the running application.
Figure 4: Retrieved SpaceX Launch Data
Get Started in Just 10 Minutes
Very little code is required to consume REST services from an Eclipse RCP client. Once this infrastructure is set up, you can start using the power of a modular user interface to leverage microservices architectures across the entire stack.
The easiest way to start is to clone the GitHub repository and run the code. It shouldn’t take longer than 10 minutes to get things running.
I’d like to thank Scott Lewis and the Eclipse Communication Framework team for doing the work that makes all of this possible. If it seems a bit too easy, remember all the effort that went into making that easy.
About the Author
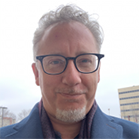