Eclipse Dirigible
Overview
Eclipse Dirigible is an open source cloud development platform, part of the Eclipse Foundation and the top-level Eclipse Cloud Development project. The ultimate goal of the platform is to provide software developers with the right toolset for building, running, and operating business applications in the cloud. To achieve this goal, Dirigible provides both independent Design Time and Runtime components.
Mission
Nowadays, providing a full-stack application development platform is not enough. Building and running on top of it has to be fast and smooth! Having that in mind, slow and cumbersome “Build”, “CI”, and “Deployment” processes have a direct impact on development productivity. In this line of thought, it isn’t hard to imagine that the Java development model for web applications doesn’t fit in the cloud world. Luckily, one of the strongest advantages of Dirigible comes at hand - the In-System Development model. Right from the early days of Dirigible, it was clear that it is going to be the platform for Business Applications Development in the cloud and not just another general purpose IDE in the browser. The reason for that decision is pretty simple - “One size doesn’t fit all”! Making a choice between providing “In-System Development” in the cloud and adding support for a new language (Java, C#, PHP, …), is really easy. The new language doesn’t really add much to the uniqueness and usability of the platform, as the In-System development model does!
Architecture
The goal of the In-System development model is to ultimately change the state of the system while it’s up and running, without affecting the overall performance and without service degradation. You can easily think of several such systems like Programmable Microcontrollers, Relational Database Management Systems, ABAP. As mentioned earlier, Dirigible provides a suitable design time and runtime for that, so let’s talk a little bit about the architecture. The Dirigible stack is pretty simple:
The building blocks are:
- Application Server (provided)
- Runtime (built-in)
- Engine(s) - (Rhino/Nashorn/V8)
- Repository - (fs/database)
- Design Time (built-in)
- Web IDE (workspace/database/git/… perspective)
- Applications (developed)
- Application (database/rest/ui)
- Application (indexing/messaging/job)
- Application (extensionpoint/extension)
- …
Enterprise JavaScript
The language of choice in the Dirigible business application platform is JavaScript! But why JavaScript? Why not Java? Is it mature enough, is it scalable, can it satisfy the business application needs? The answer is: It sure does! The code that is being written is similar to Java. The developers can write their business logic in a synchronous fashion and can leverage a large set of Enterprise JavaScript APIs. For heavy loads, the Dirigible stack performs better than the NodeJS due to multithreading of the underlying JVM and the application server, and using the same V8 engine underneath.
Examples
-
Request/Response API
var response = require('http/v3/response'); response.println("Hello World!"); response.flush(); response.close();
-
Database API:
var database = require('db/v3/database'); var response = require('http/v3/response'); var connection = database.getConnection(); try { var statement = connection.prepareStatement("select * from MY_TABLE where MY_PATH like ?"); var i = 0; statement.setString(++i, "%"); var resultSet = statement.executeQuery(); while (resultSet.next()) { response.println("[path]: " + resultSet.getString("MY_PATH")); } resultSet.close(); statement.close(); } catch(e) { console.trace(e); response.println(e.message); } finally { connection.close(); } response.flush(); response.close();
The provided Enterprise JavaScript APIs leverage some of the mature Java specifications and de facto standards (e.g. JDBC, Servlet, CMIS, ActiveMQ, File System, Streams, etc.). Eliminating the build process (due to the lack of compilation) and at the same time exposing proven frameworks (that does the heavy lifting), results in having the perfect environment for in-system development of business applications, with close to “Zero Turn-Around-Time”. In conclusion, the Dirigible platform is really tailored to the needs of Business Application Developers.
Getting Started
-
Download
- Get the latest release from: http://download.eclipse.org/dirigible
- The latest master branch can be found at: https://github.com/eclipse/dirigible
- Download the latest Tomcat 8.x from: https://tomcat.apache.org/download-80.cgi
NOTE: You can use the try out instance, that is available at http://dirigible.eclipse.org and skip through the Develop section
-
Start
- Put the ROOT.war into the
${tomcat-dir}/webapps
directory - Execute
./catalina.sh start
from the${tomcat-dir}/bin
directory
- Put the ROOT.war into the
-
Login
- Open: http://localhost:8080
- Log in with the default
dirigible/dirigible
credentials
-
Develop
-
Project
-
Create a project
- Click
+ -> Project
- Click
-
-
Database table
-
Generate a Database Table
- Right-click
New > Generate > Database table
- Right-click
-
Edit the
students.table
definition{ "name": "Students", "type": "TABLE", "columns": [{ "name": "ID", "type": "INTEGER", "primaryKey": "true" }, { "name": "FIRST_NAME", "type": "VARCHAR", "length": "50" }, { "name": "LAST_NAME", "type": "VARCHAR", "length": "50" }, { "name": "AGE", "type": "INTEGER" }] }
-
Publish
- Right-click the project and select
Publish
-
Explore
- The database scheme can be explored from the Database perspective
- Click
Window > Open Perspective > Database
- Insert some sample data
insert into students values(1, 'John', 'Doe', 25)
insert into students values(2, 'Jane', 'Doe', 23)
Note: The perspectives are available also from the side menu
NOTE: The auto publish function is enabled by default
-
- REST service
-
- Generate a Hello World service
- Right-click
New > Generate > Hello World
- Edit the
students.js
service - Explore
- The
student.js
service is accessible through thePreview
view
var database = require('db/v3/database'); var response = require('http/v3/response'); var students = listStudents(); response.println(students); response.flush(); response.close(); function listStudents() { let students = []; var connection = database.getConnection(); try { var statement = connection.prepareStatement("select * from STUDENTS"); var resultSet = statement.executeQuery(); while (resultSet.next()) { students.push({ 'id': resultSet.getInt('ID'), 'firstName': resultSet.getString('FIRST_NAME'), 'lastName': resultSet.getString('LAST_NAME'), 'age': resultSet.getInt('AGE') }); } resultSet.close(); statement.close(); } catch(e) { console.error(e); response.println(e.message); } finally { connection.close(); } return students; }
- Create a UI
- Generate a HTML5 (AngularJS) page
- Right-click
New > Generate > HTML5 (AngularJS)
- Edit the page
NOTE: All backend services are up and running after save/publish, due to the In-System Development
<!DOCTYPE html> <html lang="en" ng-app="page"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta name="description" content=""> <meta name="author" content=""> <link type="text/css" rel="stylesheet" href="/services/v3/core/theme/bootstrap.min.css"> <link type="text/css" rel="stylesheet" href="/services/v3/web/resources/font-awesome-4.7.0/css/font-awesome.min.css"> <script type="text/javascript" src="/services/v3/web/resources/angular/1.4.7/angular.min.js"></script> <script type="text/javascript" src="/services/v3/web/resources/angular/1.4.7/angular-resource.min.js"></script> </head> <body ng-controller="PageController"> <div> <div class="page-header"> <h1>Students</h1> </div> <div class="container"> <table class="table table-hover"> <thead> <th>Id</th> <th>First Name</th> <th>Last Name</th> <th>Age</th> </thead> <tbody> <tr ng-repeat="student in students"> <td>{{student.id}}</td> <td>{{student.firstName}}</td> <td>{{student.lastName}}</td> <td>{{student.age}}</td> </tr> </tbody> </table> </div> </div> <script type="text/javascript"> angular.module('page', []); angular.module('page').controller('PageController', function ($scope, $http) { $http.get('../../js/university/students.js') .success(function(data) { $scope.students = data; }); }); </script> </body> </html>
-
“What’s next?”
The In-System Development model provides the business application developers with the right toolset for rapid application development. By leveraging a few built-in templates and the Enterprise JavaScript API, whole vertical scenarios can be set up in several minutes. With the close to Zero Turn-Around-Time, changes in the backend can be made and applied on the fly, through an elegant Web IDE. The perfect fit for your digital transformation!
The goal of the Dirigible platform is clear - ease the developers as much as possible and let them concentrate on the development of critical business logic.
So, what’s next? Can I provide my own set of templates? Can I expose a new Enterprise JavaScript API? Can I provide a new perspective/view? Can I build my own Dirigible stack? Can it be integrated with the services of my cloud provider?
To all these questions, the answer is simple: Yes, you can do it!
Resources
- Site: http://www.dirigible.io
- Help: http://www.dirigible.io/help/
- API: http://www.dirigible.io/api/
- YouTube: https://www.youtube.com/c/dirigibleio
- Facebook: https://www.facebook.com/dirigible.io
- Twitter: https://twitter.com/dirigible_io
Enjoy!
About the Author
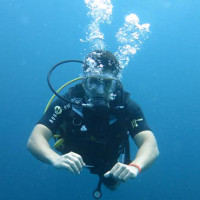