Introducing Jersey to the Eclipse Community
Most of the Java EE projects have already transitioned to the Eclipse Foundation under the Jakarta EE umbrella of projects. One of those projects is Eclipse Jersey, which is a RESTful Web services framework and reference implementation of the JAX-RS Specification. We announced the initial contribution of Jersey a few weeks ago. Since then, we saw an increase of community interest in Jersey. We registered a number of filed ideas for enhancements, discovered issues, and received pull requests from both individuals and industry. All of these are very welcome.
Jersey is used in Eclipse GlassFish and Weblogic application servers, and it is often understood to be an "enterprise" framework that needs full application server with CDI, EJB, and bean validation support, or at least a servlet container, such as Tomcat. Sure, Jersey can leverage integration with other Java EE/Jakarta EE projects, but there is more. Jersey comes with an ability to deploy JAX-RS application in a Java SE environment; it provides a container extension module that enables support for using a variety of frameworks that only understand the HTTP protocol. This is useful in the microservices world. Using Jersey, you can create a simple micro application that is capable of running inside a Docker container with just a JDK installed!
Let’s create an application that accepts queries and provides answers. First, we define the maven dependencies. Note the last hk2 dependency which is needed since Jersey 2.26:
<dependency> <groupId>org.glassfish.jersey.core</groupId> <artifactId>jersey-common</artifactId> </dependency> <dependency> <groupId>org.glassfish.jersey.core</groupId> <artifactId>jersey-client</artifactId> </dependency> <dependency> <groupId>org.glassfish.jersey.core</groupId> <artifactId>jersey-server</artifactId> </dependency> <dependency> <groupId>org.glassfish.jersey.inject</groupId> <artifactId>jersey-hk2</artifactId> </dependency>
For simplicity, we can use the HTTP server from the JDK so as not to bring other dependencies, such as the widely used Eclipse Grizzly. (Other deployment options are described in the Jersey User Guide, which has yet to be transitioned to the Eclipse Foundation):
<dependency> <groupId>org.glassfish.jersey.containers</groupId> <artifactId>jersey-container-jdk-http</artifactId> </dependency>
We would like to send popular JSON, so we add a dependency for it:
<dependency> <groupId>org.glassfish.jersey.media</groupId> <artifactId>jersey-media-json-binding</artifactId> </dependency>
We define the entity to be sent as an answer and the resource that sends responses for the ultimate question requests:
public class Answer { public Answer(String answer) { this.answer = answer; } public String answer; } @Path("/question") public class QuestionResource { public static final String RESPONSE = "42"; @GET @Path("ultimate") @Produces(MediaType.APPLICATION_JSON) public Answer getResponse() { return new Answer(RESPONSE); } }
We start the JDK HTTP Server on port 8080 with the QuestionResource
registered. We also want the entity to be wired as JSON, so we use JsonBindingFeature
:
URI base_uri = UriBuilder.fromUri("http://localhost/").port(8080).build();
ResourceConfig config = new ResourceConfig(QuestionResource.class, JsonBindingFeature.class);
JdkHttpServerFactory.createHttpServer(base_uri, config);
At this point, the Jersey application is up. We can use cURL, or even better, the JAX-RS Client (implemented by Jersey of course – and we have defined the maven org.glassfish.jersey.core:jersey-client
dependency for it already):
System.out.println(ClientBuilder.newClient().target(BASE_URI).path("question/ultimate").request().get(String.class));
And we should see the JSON response:
{"answer":"42"}
The ability to run the JAX-RS application in the Java SE environment is a new feature in the planned Eclipse JAX-RS 2.2 release.
JAX-RS is evolving fast. There are already plans for 3.0, which Jersey needs to reflect. Also there are Eclipse MicroProfile features that Jersey should either implement or integrate with. And JDK 9/10/11 need to be fully supported. There is a lot of work for the future and we are looking forward to hearing from the community. Your feature proposals, bug reports, and pull requests are very much appreciated!
About the Author
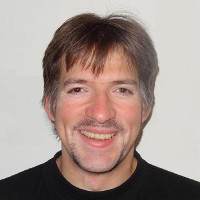